Qwen 3 Breakdown: What’s New & How It Performs
Explore Alibaba's latest AI model, Qwen 3, featuring hybrid reasoning capabilities and multilingual support. Discover its innovative design, performance benchmarks, and how it stands out in the competitive AI landscape.
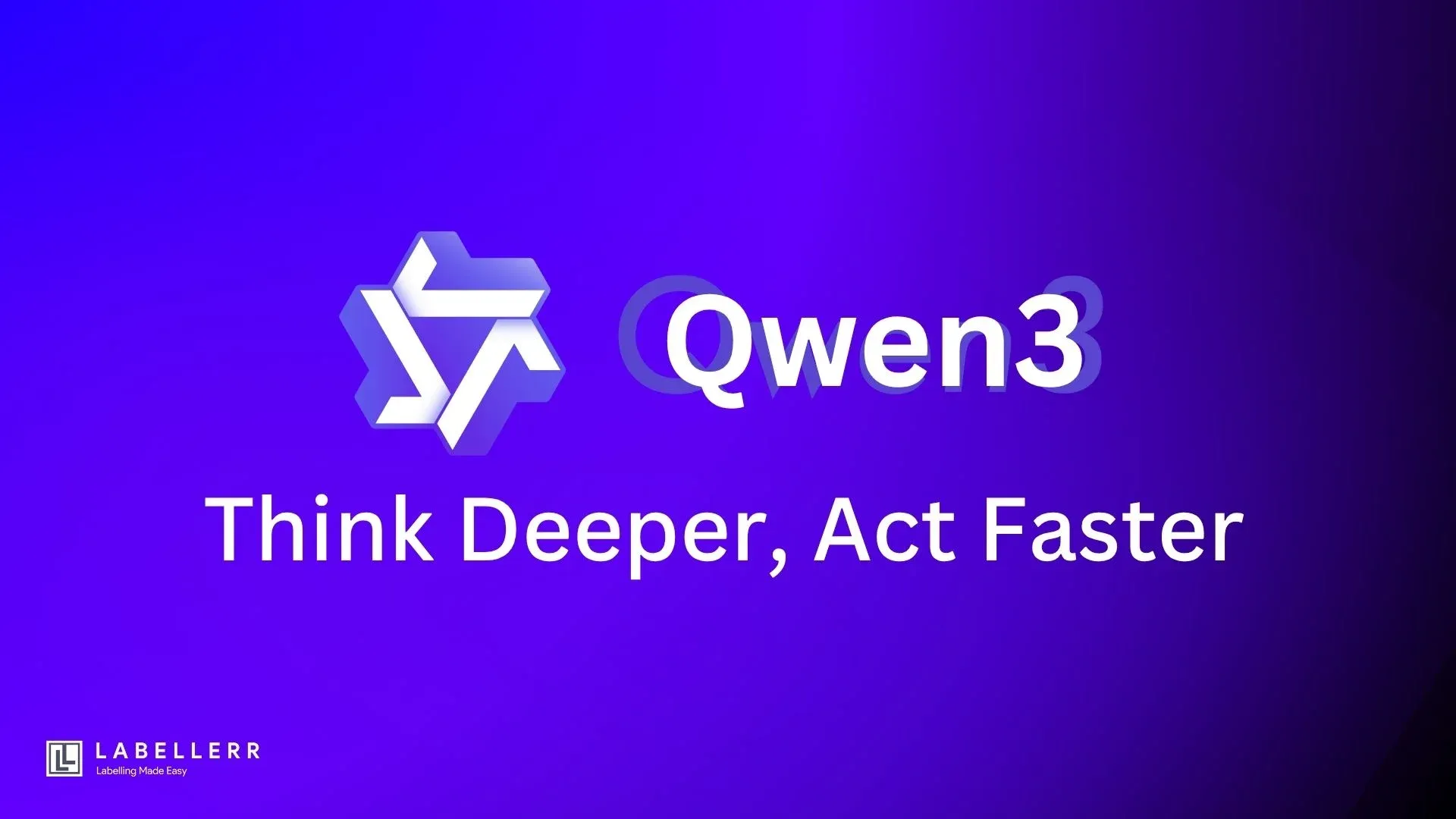
Last week, I hit a frustrating wall. I was deep into building AI agents, trying to bring some ambitious ideas to life using smolAgents and similar minimalist frameworks.
My goal was to create agents that could truly think, solve problems, and interact intelligently. But my prototypes kept stumbling.
They'd misunderstand user requests, get lost in multi-step coding challenges, and pretty much give up when faced with anything not in English.
I needed a more powerful, open-source LLM, one with strong reasoning capabilities and solid support for agentic workflows.
That's when I discovered Qwen 3, Alibaba Cloud's new series of open-weight large language models. And honestly, it felt like everything clicked into place.
In this article, we'll dive into its innovative architecture, see how it performed in hands-on tests across various tasks (including that crucial math problem!), and explore its integration with the Agents for advanced tool use.
If you're building AI agents and looking for a robust, open-source LLM, this journey might just be what you're looking for.
What Makes Qwen 3 Special?
Qwen 3 isn't just an incremental update; it introduces some significant architectural innovations and features that are particularly exciting for building AI agents.
1. The Hybrid Reasoning Engine: Thinking vs. Non-Thinking Modes
This is a standout feature. Qwen 3 can operate in two distinct modes, giving developers fine-grained control over how the model processes information:
Non-Thinking Mode: For straightforward queries, like simple chat, quick Q&A, or summarization where speed is a priority, this mode provides fast and direct responses by bypassing the deeper reasoning steps.
# Example: Getting a quick answer messages = [{"role": "user", "content": "What's the capital of France?"}] # Here, enable_thinking would be False or omitted for default behavior text_input = tokenizer.apply_chat_template( messages, tokenize=False, add_generation_prompt=True, enable_thinking=False )
Thinking Mode: When you enable this, Qwen 3 engages in a more deliberate, step-by-step reasoning process. This is invaluable for complex tasks like solving math problems, generating intricate code, or working through logical puzzles.
The model often generates intermediate <think> blocks, showing its "chain of thought," which is great for debugging and understanding its decision-making.
# Example: Forcing the model to 'think' through a problem messages = [{"role": "user", "content": "Solve this complex logic puzzle step-by-step..."}] # When applying the chat template, you specify enable_thinking=True text_input = tokenizer.apply_chat_template( messages, tokenize=False, add_generation_prompt=True, enable_thinking=True )
You can even set a "thinking budget" to balance how much computational effort the model spends on reasoning versus response speed.
2. Efficient Power with MoE (Mixture of Experts) Architecture
Qwen 3 offers some models that use a Mixture-of-Experts (MoE) architecture. This design is a clever way to build very large models that are still practical to run.
Instead of all parts of the model (all parameters) being active for every single query, an MoE model has many "expert" sub-networks. For any given input, only a few relevant experts are activated.
Model Name | Total Parameters | Active Parameters (during inference) | Estimated VRAM (16-bit precision) |
---|---|---|---|
Qwen3-30B-A3B | 30 Billion | 3 Billion | ~24GB |
Qwen3-235B-A22B | 235 Billion | 22 Billion | ~88GB |
This sparse activation means you get the power and knowledge of a massive model but with the inference cost (speed and memory) closer to that of a much smaller model.
For example, the Qwen3-30B-A3B can run on a single high-end consumer GPU (like an RTX 4090) and has shown performance comparable to much larger, closed-source models on tasks like coding.
3. Built-in Support for Model Context Protocol (MCP)
This is a big deal for agentic workflows. Qwen 3 natively supports the Model Context Protocol (MCP). MCP is a standardized way for LLMs to interact with external tools, APIs, and databases. This enables:
- Reliable Tool Calling: The model can request to use a tool (like a calculator, a weather API, or a code interpreter) without needing complex custom parsing logic on the developer's side.
- Secure Data Access: MCP can provide a more secure way for the model to access and use data from external sources.
- Dynamic Context Injection: Tools can feed information back into the model's context seamlessly.
from qwen_agent.agents import Assistant # Qwen's own agent library # Example of setting up an MCP-enabled agent # 'uvx mcp-server-time' would be a running MCP server providing time information tools_for_agent = [ {'mcpServers': {'time_tool': 'uvx mcp-server-time'}}, # Defining an MCP tool 'code_interpreter' # A built-in tool ] my_agent = Assistant(llm_config={'model': 'Qwen3-14B'}, function_list=tools_for_agent)
This native integration simplifies building agents that can interact with the outside world effectively.
My Hands-On Evaluation of Qwen-3
Talk is cheap, so I put Qwen3-14B (a good balance of power and manageability) through a series of tests focusing on the capabilities I needed for my agents. I used the Hugging Face transformers library for these.
First, loading the model:
from transformers import AutoModelForCausalLM, AutoTokenizer model_id = "Qwen/Qwen3-14B" # Or other Qwen3 model variants tokenizer = AutoTokenizer.from_pretrained(model_id) model = AutoModelForCausalLM.from_pretrained( model_id, torch_dtype="auto", # Automatically selects precision (e.g., bfloat16) device_map="auto" # Automatically distributes model across available GPUs ) def get_qwen3_response(prompt_content, enable_thinking_mode=False, max_tokens=512): messages = [{"role": "user", "content": prompt_content}] text_input = tokenizer.apply_chat_template( messages, tokenize=False, add_generation_prompt=True, enable_thinking=enable_thinking_mode # Key for controlling reasoning mode ) model_inputs = tokenizer([text_input], return_tensors="pt").to(model.device) generated_ids = model.generate( model_inputs.input_ids, max_new_tokens=max_tokens ) generated_ids = [ output_ids[len(input_ids):] for input_ids, output_ids in zip(model_inputs.input_ids, generated_ids) ] response = tokenizer.batch_decode(generated_ids, skip_special_tokens=True)[0] return response
Now, for the tests:
Test 1: Math Reasoning (Thinking Mode On)
This was a classic agent stumbling block for me.
Prompt:
# Solve step-by-step: A train leaves NYC at 3 PM traveling at 60 mph. Another train leaves Boston at 4 PM traveling at 80 mph towards NYC. The distance between NYC and Boston is 200 miles. When do they meet?
Qwen3-14B Output (Thinking Mode Enabled):
Okay, let's break this down step by step. 1. Time Difference and Head Start: * Train 1 (NYC) leaves at 3 PM. * Train 2 (Boston) leaves at 4 PM. * Train 1 has a 1-hour head start. 2. Distance Covered by Train 1 During Head Start: * Speed of Train 1 = 60 mph * Distance = 60 miles/hour × 1 hour = 60 miles 3. Remaining Distance When Train 2 Starts: * Total distance = 200 miles * Covered by Train 1 = 60 miles * Remaining = 200 - 60 = 140 miles 4. Relative Speed: * Train 1 = 60 mph * Train 2 = 80 mph * Combined = 60 + 80 = 140 mph 5. Time to Meet After Train 2 Starts: * Time = Distance / Speed = 140 / 140 = 1 hour 6. Actual Meeting Time: * Train 2 starts at 4 PM * Meeting time = 4 PM + 1 hour = 5 PM So, the trains will meet at 5 PM.
- My Observation: Perfect! The logic was clear, the intermediate steps (like the ```` block it internally generates in thinking mode) were sound, and the final answer was correct. This was a huge improvement over simpler models.
Test 2: Code Generation
Prompt:
# Instruction: Write Python code to find all prime numbers under 100 using the Sieve of Eratosthenes algorithm. Include docstrings and comments.
Qwen3-14B Output:
def sieve_of_eratosthenes(limit): """ Finds all prime numbers less than a given limit using the Sieve of Eratosthenes. Args: limit (int): Upper bound (exclusive) to find prime numbers under. Returns: List[int]: List of prime numbers less than the limit. """ is_prime = [True] * limit is_prime[0:2] = [False, False] # 0 and 1 are not prime # Iterate over numbers up to square root of the limit # If a number is prime, mark its multiples as non-prime # This reduces the search space and improves efficiency # (Sieve optimization) import math for num in range(2, int(math.sqrt(limit)) + 1): if is_prime[num]: is_prime[num * num:limit:num] = [False] * len(is_prime[num * num:limit:num]) # Extract all prime numbers from the boolean list return [i for i, prime in enumerate(is_prime) if prime] primes_under_100 = sieve_of_eratosthenes(100) print(primes_under_100)
- My Observation: Clean, efficient, and correct. The code included the requested docstrings and comments. The use of slicing for marking multiples was good practice.
Test 3: Multilingual Translation
Prompt:
# Instruction: Translate the following sentence to Swahili: "Artificial intelligence will revolutionize global healthcare systems." Provide the translation in Swahili.
Qwen3-14B Output:
# Response: Akili bandia itabadilisha mifumo ya afya duniani kwa kiasi kikubwa. (Artificial intelligence will significantly change global health systems.)
- My Observation: I checked this with online tools and a Swahili-speaking contact. The translation was accurate and natural. Qwen 3's broad language support is a clear win.
Test 4: Long-Context Handling
Test Method:
I concatenated several sections of a lengthy open-source license agreement (the Apache 2.0 license text itself, around 10k tokens) and then asked Qwen3 to summarize key clauses related to "Limitations of Liability" and "Grant of Patent License."
Prompt (simplified):
# Instruction: Summarize the key points regarding 'Limitation of Liability' and 'Grant of Patent License' from the document above.
Qwen3-14B Result:
The model accurately identified the relevant sections and summarized them correctly, picking out key phrases like "IN NO EVENT...SHALL ANY CONTRIBUTOR...BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES" for liability and the terms under which patent licenses are granted.
# Limitation of Liability: - Contributors are not liable for any damages (direct, indirect, special, incidental, or consequential) arising from the use or inability to use the software, regardless of the legal theory (tort, contract, etc.). - This exclusion applies even if the contributor was advised of the possibility of such damages. - The only exceptions are if liability is required by applicable law (such as for deliberate or grossly negligent acts) or agreed to in writing. # Grant of Patent License: - Each contributor grants users a perpetual, worldwide, royalty-free, irrevocable patent license to use, make, sell, or distribute the contributor's code, covering only those patent claims necessarily infringed by their contribution. - This license is limited to patents directly tied to the contributed code, not unrelated patents. - If a user initiates patent litigation over the licensed software, their rights under the license (including the patent grant) are terminated. - This provision encourages open collaboration and protects users from patent claims related to contributed code.
- My Observation: It successfully navigated the long text, retained context, and extracted the correct information without hallucinating contractual terms. This is crucial for agents that might need to process large documents.
Test 5: Creative Writing
Prompt:
# Instruction: Write a haiku about the concept of quantum entanglement.
Qwen3-14B Output:
# Response: Distant twins entwine,
One state mirrors other's fate,
Spacetime wonders.
- My Observation: It nailed the 5-7-5 syllable structure and captured the essence of entanglement poetically. Impressive creative flair.
Test 6: Commonsense QA
Prompt:
# Question: Why do ice cubes float in a glass of water? # Response: Ice cubes float in water because ice is less dense than liquid water. Here's a more detailed explanation: When water freezes, its molecules arrange themselves into a rigid, open lattice structure. This crystalline structure takes up more space than the more closely packed molecules in liquid water. Because the same amount of mass (the water molecules) occupies a larger volume when it's ice, its density (mass per unit volume) decreases. Specifically, the density of ice is about 0.917 grams per cubic centimeter (g/cm³), while the density of liquid water is about 1.0 g/cm³ (at 4°C, where water is most dense). Since ice is less dense than the liquid water it's placed in, it is buoyant and floats on top.
- My Observation: A clear, accurate, and scientifically sound explanation of a common sense phenomenon.
Test 7: Tool Use via MCP
While I didn't set up a full uvx MCP server for this specific quick test, I explored how the qwen-agent library facilitates this.
The logic is straightforward. A MCP server named mcp-server-weather that provides a _current_weather(city: str) function.
# Example: Custom Weather Tool Integration with Qwen Agent from qwen_agent.agents import Assistant from qwen_agent.tools.base import BaseTool, register_tool # Imagine this tool connects to our MCP server or a direct API @register_tool('weather_tool') class MyWeatherTool(BaseTool): name = 'weather_tool' description = 'Gets the current weather for a specified city.' parameters = [{ 'name': 'city', 'type': 'string', 'description': 'The city for which to get the weather.', 'required': True }] # Simulate API call for weather data def call(self, params: str, **kwargs) -> str: import json city_name = json.loads(params).get('city', 'UnknownCity') if city_name.lower() == 'paris': return json.dumps({"city": "Paris", "temperature": "22°C", "condition": "sunny"}) return json.dumps({"city": city_name, "error": "Weather data not found"}) # Configure the agent to use this tool agent = Assistant( llm_config={'model': 'Qwen3-14B'}, function_list=['weather_tool'] ) # Agent query user_query = "What's the weather like in Paris right now?" messages = [{"role": "user", "content": user_query}] # The agent should internally decide to use 'weather_tool' # and then generate a response based on the tool's output. # Print the streaming response chunks # (current date: Wednesday, May 14, 2025, 12:45 PM IST) for response_chunk in agent.run(messages=messages): print(response_chunk)
Agent Workflow (Conceptual):
- Qwen 3 (via the Assistant agent) analyzes the query: "What's the weather like in Paris right now?"
- It identifies that the weather_tool can answer this.
- It formulates a call to weather_tool with city="Paris".
- The tool (simulated above) returns: {"city": "Paris", "temperature": "22°C", "condition": "sunny"}.
- This result is injected back into Qwen 3's context.
- Qwen 3 generates the final user-facing response: "The current weather in Paris is 22°C with sunny skies."
- My Observation: The qwen-agent library and Qwen 3's underlying capabilities make this kind of tool integration much cleaner than manual parsing. The native MCP support promises even more standardized and robust interactions.
MCP Integration
The Model Context Protocol (MCP) is a key piece of the puzzle for building truly capable AI agents. My exploration confirmed why it's so important:
- Standardized Tooling: MCP aims to replace the often brittle and error-prone methods of parsing LLM outputs to trigger tools (like using regex). It provides a structured way for models to declare their intent to use a tool and for tools to return information.
- More Secure Context Injection: When tools provide information back to the LLM, MCP can help ensure this is done in a way that minimizes risks like prompt injection or a tool overwhelming the model's context.
- Potential for Cross-Model Compatibility: Because MCP is a protocol, the idea is that an MCP-enabled tool server could, in theory, work with different LLMs that also support MCP (like some versions of Claude or potentially future GPT models if they adopt it).
A Quick Guide to Using MCP with Qwen Agent (Conceptual)
Here’s a more concrete look at how you might set up a simple MCP tool using uvx (a utility often used with MCP) and Qwen Agent.
1. Launch an MCP Server (Example: A Time Server)
You'd typically run this in a separate terminal. uvx helps create these servers.
# This command would start an MCP server that provides the current time. # The actual command might vary based on the uvx tool implementation. uvx mcp-server-time --local-timezone=Europe/Paris --port 8080 --service-name my-time-service
This server would now be listening, ready to provide time information when called via MCP.
2. Configure Your Qwen Agent to Use the MCP Server
# Example: Configure Qwen Assistant with MCP and Code Interpreter Tools from qwen_agent.agents import Assistant # Define the tools, pointing to the running MCP server agent_tools = [ { 'mcpServers': { 'current_time_tool': 'http://localhost:8080/my-time-service' # Name and URL of MCP service } }, 'code_interpreter' # Another built-in or custom tool ] # Initialize the Qwen Assistant bot = Assistant( llm_config={'model': 'Qwen3-14B'}, # Specify your Qwen3 model function_list=agent_tools )
3. Have the Agent Execute a Task Using the MCP Tool
# User asks the agent for the current time in Paris using a time tool user_messages = [{"role": "user", "content": "What time is it in Paris according to your time tool?"}] # Stream and print the agent's response as it arrives for response_part in bot.run(messages=user_messages): print(response_part.get("content", ""), end="") print() # Newline after full response
- The Assistant agent, powered by Qwen 3, would ideally:
- Understand the need for the current_time_tool.
- Make an MCP call to http://localhost:8080/my-time-service.
- Receive the time data.
- Formulate a natural language response, e.g., "The current time in Paris is 14:35 CEST."
This streamlined interaction is what makes MCP so promising for complex agentic systems.
The Verdict
Based on my testing and research into benchmarks, here's how I see Qwen3-14B comparing to some alternatives in the context of building AI agents:
Model Capability | Qwen3-14B (My Experience & Benchmarks) |
DeepSeek Coder/R1 (Focus on Code/General) |
Phi-3-mini/small (Smaller, Capable) |
Qwen 2.5 (Previous Gen) |
---|---|---|---|---|
Complex Reasoning | ★★★★★ (Thinking mode is excellent) | ★★★★☆ (Good, esp. R1) | ★★★☆☆ (Good for size) | ★★★★☆ |
Coding Ability | ★★★★☆ (Very Solid) | ★★★★★ (DeepSeek Coder excels) | ★★★☆☆ (Decent) | ★★★☆☆ |
Multilingual | 119+ languages (Excellent) | Varies, R1 good general coverage | Good, but less broad than Qwen3 | ~29 languages |
Agent/Tool Support | Native MCP (Excellent via Qwen Agent) | Via frameworks, less native integration | Via frameworks | Plugin-based, less integrated |
Openness/License | Apache 2.0 (Commercial use OK) | Apache 2.0 / Open | MIT (Commercial use OK) | Tongyi Qianwen License |
Ease of Local Run | Good for 14B (e.g., on RTX 4090) | Similar for comparable sizes | Excellent (runs on less VRAM) | 72B is very demanding |
Key Advantages of Qwen 3 for My Agentic Workflows:
- The Thinking/Non-Thinking Mode: This is a genuine differentiator for crafting nuanced agent behavior.
- MoE Efficiency: Larger Qwen 3 MoE models promise top-tier performance without insane hardware demands (e.g., Qwen3-30B-A3B).
- Native MCP Integration Potential: This future-proofs agent development, moving towards more standardized tool use. The qwen-agent library already provides a strong foundation.
- Excellent Multilingual and Long-Context: Broadens the applicability of agents significantly.
- Permissive Apache 2.0 License: Crucial for many projects, including potential commercial ones. Reports suggest Qwen 3 models often outperform older, larger models (like Qwen2.5-72B) on many tasks while being much more efficient [10][17].
Conclusion
After hours of wrestling with simpler frameworks and models, discovering and testing Qwen 3 felt like a breath of fresh air.
For developers like me, trying to build AI agents that can actually reason, solve complex problems, use tools effectively, and communicate across languages, Qwen 3 offers a powerful and accessible open-source solution.
My key takeaways:
- Reasoning Transformed: The "thinking mode" genuinely elevates the model's ability to tackle complex tasks that left my earlier smol-agent prototypes struggling.
- Practical Power: The MoE architecture in larger Qwen 3 variants means access to near state-of-the-art capabilities without needing a data center. Even the 14B dense model is remarkably capable on consumer hardware.
- Agent-Ready Ecosystem: With features like MCP support and libraries like qwen-agent, Qwen 3 is clearly built with agentic systems in mind.
Qwen 3 isn't just another LLM release; for me, it represents a significant step forward for building sophisticated, open-source AI agents. It has become the default foundation for my projects, and I'm excited to see what I can build with it next. If you're on a similar journey, I highly recommend giving Qwen 3 a serious look.
FAQs
Q1: What is Qwen 3?
A: Qwen 3 is Alibaba's latest AI model, released on April 28, 2025, featuring hybrid reasoning capabilities and support for 119 languages and dialects.
Q2: What are the key features of Qwen 3?
A: Qwen 3 offers both dense and sparse models, a 128K token context window (for most variants), and hybrid reasoning that can be toggled via the tokenizer.
Q3: How does Qwen 3 perform compared to other models?
A: Qwen 3 demonstrates competitive performance in reasoning and problem-solving tasks, rivaling models like DeepSeek's R1 and OpenAI's offerings.
Q4: Is Qwen 3 open-source?
A: Yes, Qwen 3 models are open-sourced under the Apache 2.0 license and are available on platforms like Hugging Face and ModelScope.
Q5: Where can developers access Qwen 3?
A: Developers can access Qwen 3 through chat.qwen.ai and integrate it via APIs provided on platforms like Hugging Face and ModelScope.
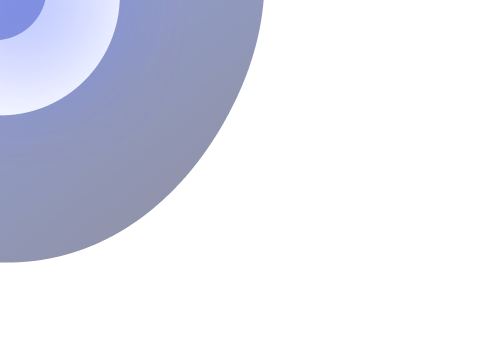
Simplify Your Data Annotation Workflow With Proven Strategies
.png)
